Advanced Frontend Frameworks
Take your frontend skills to the next level by mastering modern JavaScript frameworks. Focus on React with additional exposure to other essential frontend tools and libraries.
Course Highlights
Everything you need to master modern frontend frameworks
- 12 weeks (36 hours of instruction)
- Small classes (max 12 students)
- Focus on React with Hooks & Context API
- State management (Redux & Context API)
- Build a full-featured React application
Course Overview
Our Advanced Frontend Frameworks course is designed for developers who have a solid understanding of JavaScript and want to master modern frontend frameworks and libraries, with a primary focus on React.
Over 12 weeks, you'll learn how to build sophisticated, scalable web applications using React and its ecosystem. You'll master component architecture, state management, routing, and integration with APIs. We'll also introduce you to other popular frameworks to give you a broader perspective.
By the end of this course, you'll be able to create professional-quality React applications, understand advanced state management techniques, and have the skills needed to work as a frontend developer in modern web development teams.
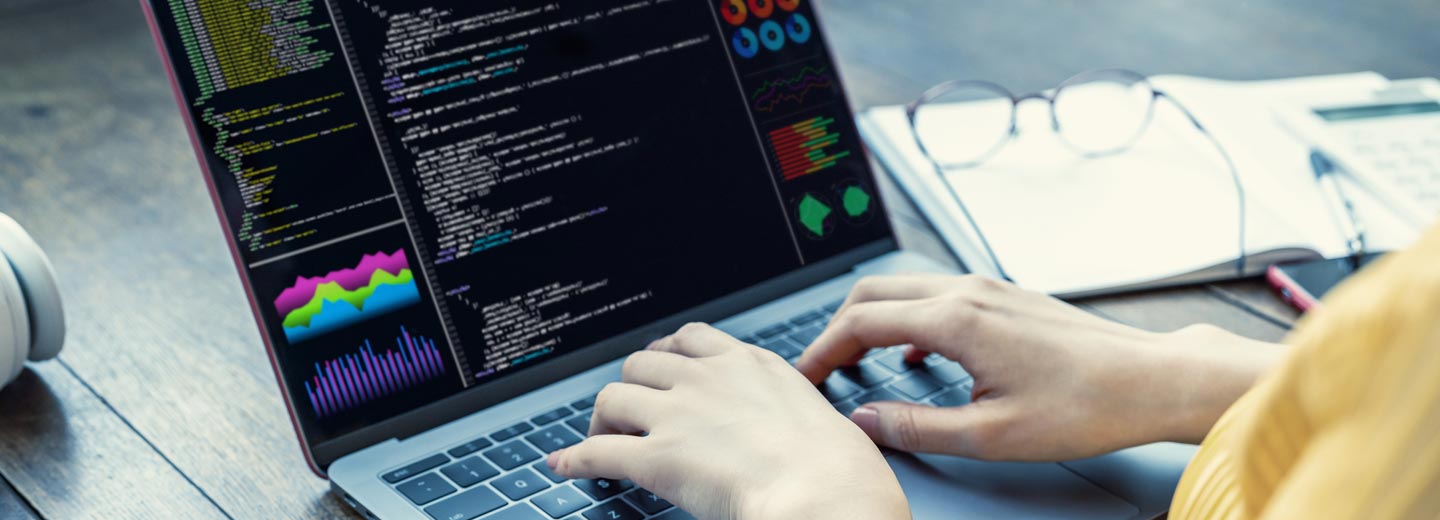
What You'll Learn
- Modern React with Hooks and functional components
- State management with Context API and Redux
- Routing and navigation in single-page applications
- API integration and asynchronous operations
- Testing and debugging React applications
- Introduction to other frameworks (Vue, Svelte)
Frameworks & Libraries You'll Learn
Master the tools that power modern web applications.
React
Primary focus of the course
Vue.js
Introduction to alternative
State Management
Redux & Context API
Testing
Unit & Integration Testing
A Glimpse of React
Here's a taste of what you'll be coding during the course.
React Functional Component with Hooks
import React, { useState, useEffect } from 'react';
const UserProfile = ({ userId }) => {
const [user, setUser] = useState(null);
const [loading, setLoading] = useState(true);
const [error, setError] = useState(null);
useEffect(() => {
const fetchUser = async () => {
try {
setLoading(true);
const response = await fetch(
`https://api.example.com/users/${userId}`
);
if (!response.ok) {
throw new Error('Failed to fetch user data');
}
const data = await response.json();
setUser(data);
setError(null);
} catch (err) {
setError(err.message);
setUser(null);
} finally {
setLoading(false);
}
};
fetchUser();
}, [userId]);
if (loading) return Loading user data...;
if (error) return Error: {error};
if (!user) return No user found;
return (
{user.name}
Email: {user.email}
Location: {user.location}
);
};
Learn how to build functional components with React Hooks for state management and side effects. This example demonstrates fetching data from an API and handling loading/error states.
Context API for State Management
// ThemeContext.js
import React, { createContext, useState, useContext } from 'react';
const ThemeContext = createContext();
export const ThemeProvider = ({ children }) => {
const [theme, setTheme] = useState('light');
const toggleTheme = () => {
setTheme(prevTheme =>
prevTheme === 'light' ? 'dark' : 'light'
);
};
return (
{children}
);
};
export const useTheme = () => useContext(ThemeContext);
// App.js
import { ThemeProvider } from './ThemeContext';
import Header from './Header';
import Content from './Content';
const App = () => {
return (
);
};
// Header.js
import { useTheme } from './ThemeContext';
const Header = () => {
const { theme, toggleTheme } = useTheme();
return (
My App
);
};
Master global state management with React's Context API. This example shows how to create a theme context that can be accessed by any component in your application.
Who Is This Course For?
Our Advanced Frontend Frameworks course is ideal for:
JavaScript Developers
If you're comfortable with JavaScript and want to learn modern frontend frameworks to create more complex, interactive applications, this course is perfect for you. You'll build on your existing JavaScript knowledge to master component-based architecture.
Career Advancers
Looking to level up your career in web development? React is one of the most in-demand skills in the industry. This course will give you the expertise needed to apply for intermediate to senior frontend positions at companies using modern tech stacks.
Project Builders
If you want to build sophisticated web applications, whether for your own projects, startup ideas, or client work, this course will give you the tools and knowledge to create professional-quality applications with modern architecture and best practices.
Course Curriculum
Our comprehensive curriculum will take you from JavaScript to building complex React applications.
Modern Frontend Development Overview
Introduction to modern frontend development landscape and the role of frameworks.
- • Evolution of frontend development
- • Component-based architecture
- • Virtual DOM and its benefits
- • Overview of popular frameworks
Introduction to React & Component Architecture
Learn the fundamentals of React and component-based development.
- • React core concepts and philosophy
- • Setting up a React project (Create React App)
- • JSX syntax and expressions
- • Component types and composition
React Hooks & Functional Components
Master modern React with hooks and functional components.
- • useState for local state management
- • useEffect for side effects
- • Custom hooks for reusable logic
- • useRef, useMemo, and useCallback for optimization
State Management with Context API & Redux
Learn different approaches to manage state in complex applications.
- • React Context API for global state
- • Introduction to Redux and its principles
- • Redux Toolkit for modern Redux development
- • When to use each state management solution
Routing & Navigation in Single Page Applications
Implement client-side routing for multi-page experiences.
- • React Router setup and configuration
- • Route parameters and query strings
- • Nested routes and layouts
- • Protected routes and authentication
API Integration & Data Fetching
Learn how to connect your frontend to backend services.
- • Working with REST APIs
- • Fetch API vs Axios
- • Handling loading and error states
- • Data caching and optimization
Forms & User Input
Master form handling and validation in React applications.
- • Controlled vs uncontrolled components
- • Form state management
- • Validation and error handling
- • Form libraries (Formik, React Hook Form)
Performance Optimization & Best Practices
Learn techniques for building fast and efficient React applications.
- • Memo, useMemo, and useCallback optimization
- • React.lazy and code splitting
- • Performance measurement and debugging
- • Common optimization patterns
Introduction to Vue.js & Other Frameworks
Explore alternative frameworks to broaden your perspective.
- • Vue.js core concepts and setup
- • Comparing React and Vue approaches
- • Overview of Svelte and its advantages
- • Choosing the right framework for your project
Testing & Debugging React Applications
Ensure code quality with testing and efficient debugging.
- • Jest and React Testing Library
- • Component testing strategies
- • Testing hooks and context
- • React DevTools for debugging
Final Project: Full-featured React Application
Apply everything you've learned to build a comprehensive web application.
- • Project planning and architecture
- • Component design and reusability
- • State management implementation
- • API integration and data handling
- • Routing and navigation
- • Testing and performance optimization
- • Deployment and CI/CD
Meet Your Instructor
Learn from experienced professionals who are passionate about teaching.
Bogdan Tajikistanovich
Lead Frameworks Instructor
Bogdan brings over 12 years of experience in frontend development, specializing in modern JavaScript frameworks like React, Vue, and Angular. He has worked with startups and established companies across Europe, leading teams to build complex, scalable web applications.
Prior to joining Frontend Masters Cyprus, Bogdan was a Technical Lead at several startups in Berlin and London, where he architected frontend solutions and mentored junior developers. His teaching approach combines theoretical concepts with practical, real-world examples from his extensive industry experience.
Pricing & Upcoming Dates
Invest in your future with our comprehensive Advanced Frontend Frameworks course.
Course Details
All-inclusive price
- 12 weeks of instruction (36 hours total)
- Small class size (maximum 12 students)
- Comprehensive course materials
- Certificate of completion
- Ongoing support during the course
- Access to recorded sessions
* Payment plans available - spread the cost over 3 months with no additional fees
* 5% discount for full upfront payment
* Group discounts available for 3+ enrollments
* 10% discount for JavaScript course graduates
Upcoming Course Dates
Evening Session
Enrolling NowJuly 10 - September 25, 2025
Tuesday & Thursday, 6:30 PM - 8:00 PM
6 spots remaining
Weekend Session
Enrolling NowJuly 12 - September 27, 2025
Saturday, 10:00 AM - 1:00 PM
8 spots remaining
Morning Session
Coming SoonSeptember 8 - November 24, 2025
Monday & Wednesday, 10:00 AM - 11:30 AM
Registration opens August 1, 2025
* Custom corporate training schedules available upon request
* Online options available for students outside of Limassol/Nicosia
Frequently Asked Questions
Get answers to common questions about our Advanced Frontend Frameworks course.
How much JavaScript knowledge do I need?
You should have a solid foundation in JavaScript before taking this course. You should be comfortable with:
- Core JavaScript concepts (variables, functions, objects, arrays)
- ES6+ features (arrow functions, destructuring, promises)
- DOM manipulation
- Asynchronous JavaScript (promises, async/await)
Our JavaScript Essentials course provides all these prerequisites, but if you've learned these skills elsewhere, that's fine too. If you're unsure, feel free to contact us for an assessment or recommendation.
Why focus mainly on React instead of other frameworks?
We focus primarily on React for several reasons:
- React has the largest market share among frontend frameworks, meaning more job opportunities
- It has a robust ecosystem of libraries and tools
- Its component-based architecture teaches fundamental concepts that transfer to other frameworks
- There's high demand for React developers in Cyprus and internationally
That said, we do introduce other frameworks like Vue.js to give you a broader perspective. The core concepts you learn with React (components, state management, etc.) will help you pick up other frameworks more easily in the future.
What kind of projects will I build during the course?
Throughout the course, you'll work on several projects of increasing complexity:
- Single-component applications like interactive widgets
- Multi-component applications with state management
- A full-featured single-page application (SPA) with routing and API integration
For your final project, you'll build a substantial application that could be:
- An e-commerce platform
- A social media dashboard
- A content management system
- Or a custom project based on your interests
This final project will showcase all the skills you've learned and serve as an impressive portfolio piece for potential employers.
Will this course prepare me for a job as a React developer?
Yes! This course is specifically designed to prepare you for real-world jobs using React and modern frontend technologies. By the end of the course, you'll have:
- A strong foundation in React development
- Experience with industry best practices and design patterns
- Knowledge of common tools in the React ecosystem
- Portfolio projects to showcase your skills
- Experience with collaborative development workflows
Many of our graduates have successfully transitioned into React developer roles or have significantly upgraded their existing positions. We also provide career support including resume reviews, interview preparation, and connections with local employers looking for React talent.
How does this course compare to online tutorials or courses?
While there are many online resources for learning React, our course offers several advantages:
- Direct access to experienced instructors who can answer questions and provide personalized guidance
- A structured, comprehensive curriculum that builds progressively
- Real-time feedback on your code and projects
- The accountability and motivation that comes with scheduled classes
- A community of fellow learners to collaborate with
- Industry connections specific to the Cyprus market
Many of our students have tried learning from online tutorials before joining us and found that the structured approach and personal guidance accelerated their learning significantly.
Ready to Master Modern Frontend Frameworks?
Enroll in our Advanced Frontend Frameworks course and take your development skills to the next level.
Enrollment Form
Have Questions?
We're happy to answer any questions you may have about our Advanced Frontend Frameworks course or any of our other offerings.
- Response within 24 hours
- Schedule a free consultation call
- Attend a free intro session
Why Learn React & Modern Frameworks?
Learning React and modern frontend frameworks is essential for anyone serious about a career in web development. Here's why:
- React developers command 25-40% higher salaries
- Most in-demand frontend framework worldwide
- Growing ecosystem with transferable skills
Master Modern Frontend Frameworks with Cyprus's Premier Development Course
In today's competitive tech landscape, knowing the fundamentals of HTML, CSS, and JavaScript is just the beginning. Modern web development demands expertise in frameworks and libraries that enable developers to build sophisticated, interactive applications efficiently.
Our Advanced Frontend Frameworks course is designed to bridge the gap between basic web development skills and the professional-level expertise that employers in Cyprus and beyond are seeking. With a primary focus on React—the most in-demand frontend library worldwide—this course equips you with the skills to create complex, scalable web applications using industry best practices.
The tech sector in Cyprus has seen remarkable growth in recent years, with both local companies and international firms establishing presence on the island. This growth has created unprecedented demand for developers proficient in modern frontend frameworks, particularly React. By mastering these technologies, you position yourself at the forefront of this exciting field, opening doors to remote work opportunities, local tech positions, and freelance projects.
What sets our course apart is our comprehensive, project-based approach. Rather than simply teaching syntax and theory, we guide you through building actual applications that solve real-world problems. Our instructor brings over a decade of industry experience to the classroom, sharing insights, best practices, and common pitfalls that can only come from years of professional development work.
Whether you're looking to advance your career, transition into web development from another field, or enhance your skillset as a freelancer, our Advanced Frontend Frameworks course provides the perfect blend of theoretical knowledge and practical experience to help you achieve your goals. Join us and become part of Cyprus's growing community of skilled React developers!
Ready to Master Modern Frontend Frameworks?
Enroll in our Advanced Frontend Frameworks course today and transform your development career.