JavaScript Essentials
Master modern JavaScript to create dynamic, interactive websites. This comprehensive course covers core concepts, DOM manipulation, and essential ES6+ features.
Course Highlights
Everything you need to master JavaScript fundamentals
- 10 weeks (30 hours of instruction)
- Small classes (max 12 students)
- Modern ES6+ JavaScript
- Build interactive web applications
- Requires basic HTML & CSS knowledge
Course Overview
Our JavaScript Essentials course is designed for those who have a foundational understanding of HTML and CSS and are ready to add interactivity to their websites. JavaScript is the programming language of the web, and mastering it is essential for any aspiring frontend developer.
Over 10 weeks, you'll learn how to use JavaScript to manipulate the DOM, handle events, work with data, and create interactive user experiences. We focus on modern ES6+ features and best practices that align with today's industry standards.
By the end of this course, you'll be able to build dynamic web applications, understand how to work with APIs, and have the skills needed to progress to more advanced frontend frameworks like React or Vue.
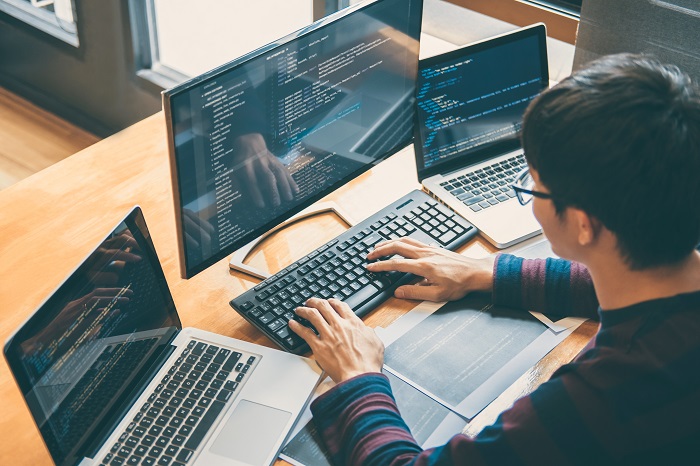
What You'll Learn
- JavaScript fundamentals and core concepts
- DOM manipulation and event handling
- Modern ES6+ features (arrow functions, destructuring, etc.)
- Working with APIs and asynchronous JavaScript
- Error handling and debugging techniques
- Building an interactive web application
A Taste of JavaScript
Here's a glimpse of what you'll be learning and creating during the course.
Basic DOM Manipulation
// Select elements
const button = document.querySelector('#myButton');
const output = document.querySelector('#output');
// Add event listener
button.addEventListener('click', () => {
// Change content
output.textContent = 'Button was clicked!';
// Add a class for styling
output.classList.add('highlight');
// Create a new element
const timestamp = document.createElement('div');
timestamp.textContent = new Date().toLocaleTimeString();
// Add it to the DOM
output.appendChild(timestamp);
});
You'll learn how to select elements, listen for events, manipulate content, and dynamically create new elements to create interactive user experiences.
Modern ES6+ Features
// Default parameters
const greet = (name = 'Guest') => `Hello, ${name}!`;
// Destructuring
const person = { name: 'Sofia', age: 28, city: 'Limassol' };
const { name, city } = person;
// Spread operator
const numbers = [1, 2, 3];
const moreNumbers = [...numbers, 4, 5];
// Async/await for API calls
async function fetchUserData(userId) {
try {
const response = await fetch(`https://api.example.com/users/${userId}`);
const data = await response.json();
return data;
} catch (error) {
console.error('Error fetching user data:', error);
}
}
You'll master modern JavaScript features that make your code more concise, readable, and powerful, preparing you for professional development environments.
Who Is This Course For?
Our JavaScript Essentials course is ideal for:
HTML & CSS Developers
If you're comfortable with HTML and CSS (whether from our Fundamentals course or elsewhere) and ready to add interactivity to your websites, this course is the perfect next step in your learning journey.
Aspiring Frontend Developers
JavaScript is essential for anyone looking to build a career in frontend development. This course will give you the programming fundamentals needed to progress to more advanced frameworks and libraries.
Designers & Content Creators
If you work in digital design or content creation and want to enhance your skills with interactive elements, animations, and dynamic content, JavaScript will significantly expand your capabilities.
Course Curriculum
Our comprehensive curriculum will take you from JavaScript basics to building complex interactive applications.
JavaScript Fundamentals & Syntax
Introduction to JavaScript, its role in web development, and core syntax elements.
- • JavaScript's role in the modern web
- • Setting up a development environment
- • Basic syntax and code structure
- • Working with the browser console
Variables, Data Types & Operators
Learn about different data types in JavaScript and how to work with them.
- • Variables, constants, and scope
- • Primitive types: strings, numbers, booleans
- • Complex types: arrays and objects
- • Operators and expressions
Control Flow, Functions & Scope
Master control structures and understand how to create reusable code with functions.
- • Conditional statements (if, else, switch)
- • Loops (for, while, for...of, for...in)
- • Function declarations and expressions
- • Understanding scope and closures
Arrays, Objects & the DOM
Work with complex data structures and learn to interact with HTML elements.
- • Array methods and operations
- • Creating and manipulating objects
- • DOM selection and traversal
- • Modifying element content and attributes
Events & Event Handling
Learn how to make your pages interactive by responding to user actions.
- • Understanding the event model
- • Common events (click, submit, load, etc.)
- • Event delegation and propagation
- • Building interactive UI components
ES6+ Features
Explore modern JavaScript features that improve code readability and functionality.
- • Arrow functions and template literals
- • Destructuring assignments
- • Spread and rest operators
- • Default parameters and modules
Asynchronous JavaScript
Understand how to work with asynchronous operations in JavaScript.
- • Callbacks and callback hell
- • Promises and promise chaining
- • Async/await syntax
- • Error handling in async code
Fetch API & Working with JSON
Learn to communicate with servers and external APIs to get and send data.
- • Understanding HTTP and APIs
- • Using the Fetch API
- • Working with JSON data
- • Practical API integration examples
Error Handling & Debugging
Master techniques for finding and fixing errors in your JavaScript code.
- • Common JavaScript errors
- • Try...catch statements
- • Using browser developer tools
- • Debugging strategies and techniques
Final Project: Interactive Web Application
Apply everything you've learned to build a complete interactive web application.
- • Project planning and architecture
- • Implementing core functionality
- • API integration and data management
- • Testing and deployment
Meet Your Instructor
Learn from experienced professionals who are passionate about teaching.
Alexei Kazakhstanov
Lead JavaScript Instructor
Alexei brings over 10 years of experience in frontend development, having worked with companies ranging from startups to large enterprises. He specializes in JavaScript and modern frameworks, with a particular focus on creating performant, accessible web applications.
Prior to joining Frontend Masters Cyprus, Alexei worked as a Senior Frontend Developer at ByteSpark, where he led the development of complex web applications and mentored junior developers. His teaching approach combines theoretical concepts with practical, real-world examples that help students truly understand JavaScript.
What Our Students Say
Hear from graduates who have completed our JavaScript Essentials course.
Marika Uzbekistanova
Frontend Developer
"The JavaScript course was intensive but incredibly rewarding. The instructors were patient and took the time to ensure everyone understood complex concepts before moving on. After completing this course, I was able to build my own portfolio website with interactive features that helped me land my first development job."
Gorislav Tajikistanovich
Computer Science Student
"I took this course to supplement my computer science degree. While university taught me programming theory, this course gave me practical JavaScript skills that employers actually want. The hands-on approach and real-world projects were invaluable. I now feel confident applying for internships with the portfolio I built during the course."
Nikolai Kyrgyzstansky
UX Designer
"As a designer, I wanted to understand how to implement my designs and create interactive prototypes. This course was perfect - it taught me enough JavaScript to bring my UX designs to life. Now I can create functional prototypes that demonstrate interactions, which has made me much more valuable in my design role."
Pricing & Upcoming Dates
Invest in your future with our comprehensive JavaScript course.
Course Details
All-inclusive price
- 10 weeks of instruction (30 hours total)
- Small class size (maximum 12 students)
- Comprehensive course materials
- Certificate of completion
- Ongoing support during the course
- Access to recorded sessions
* Payment plans available - spread the cost over 3 months with no additional fees
* 5% discount for full upfront payment
* Group discounts available for 3+ enrollments
* 10% discount for HTML & CSS course graduates
Upcoming Course Dates
Morning Session
Enrolling NowJune 5 - August 7, 2025
Monday & Wednesday, 10:00 AM - 11:30 AM
5 spots remaining
Evening Session
Enrolling NowJune 6 - August 8, 2025
Tuesday & Thursday, 6:30 PM - 8:00 PM
7 spots remaining
Weekend Session
Coming SoonJuly 12 - September 13, 2025
Saturday, 10:00 AM - 1:00 PM
Registration opens June 1, 2025
* Custom corporate training schedules available upon request
* Online options available for students outside of Limassol/Nicosia
Frequently Asked Questions
Get answers to common questions about our JavaScript Essentials course.
How much HTML & CSS knowledge do I need?
You should have a basic understanding of HTML and CSS before starting this course. You should be comfortable with:
- Creating HTML documents with proper structure
- Using common HTML elements (headings, paragraphs, links, images)
- Basic CSS styling and selectors
- Understanding the box model and simple layouts
Our HTML & CSS Fundamentals course provides all these prerequisites, but if you've learned these skills elsewhere, that's fine too. If you're unsure, feel free to contact us for an assessment.
Is this course suitable for complete beginners to programming?
If you've never programmed before, this course might be challenging but is still achievable with dedication. JavaScript is often people's first programming language, and we design our curriculum to accommodate those new to programming concepts.
However, if you're completely new to both HTML/CSS and programming, we recommend taking our HTML & CSS Fundamentals course first. This provides a gentler introduction to web technologies before diving into JavaScript.
What will I be able to build after completing this course?
By the end of this course, you'll be able to:
- Create interactive web pages with dynamic content
- Build forms with validation and user feedback
- Create UI components like tabs, modals, sliders, and dropdowns
- Fetch and display data from APIs
- Implement client-side filtering and sorting of data
- Create simple single-page applications
Your final project will be a complete interactive web application that demonstrates these skills and can serve as a portfolio piece for potential employers.
Will this prepare me for learning React or other frameworks?
Absolutely! A solid understanding of JavaScript is essential before learning any JavaScript framework like React, Vue, or Angular. This course specifically focuses on modern JavaScript features (ES6+) that are heavily used in these frameworks.
After completing this course, you'll have the necessary foundation to progress to our Advanced Frontend Frameworks course, which covers React in depth along with an introduction to other popular frameworks.
How much practice time should I allocate outside of class?
We recommend allocating at least 4-6 hours per week for practice and homework outside of the scheduled classes. Programming is a skill that requires practice, and the more time you can dedicate to coding, the more comfortable you'll become.
We provide weekly coding challenges and exercises that reinforce the concepts taught in class. Additionally, our instructors are available during office hours and via our online community platform to help with any questions that arise during your practice time.
Ready to Master JavaScript?
Enroll in our JavaScript Essentials course and take the next step in your web development journey.
Enrollment Form
Have Questions?
We're happy to answer any questions you may have about our JavaScript Essentials course or any of our other offerings.
- Response within 24 hours
- Schedule a free consultation call
- Attend a free intro session
JavaScript: The Language of the Web
JavaScript is the programming language that powers the modern web. Nearly every interactive element you encounter online is built with JavaScript.
- The most in-demand programming language for web developers
- Essential skill for frontend, backend, and full-stack development
- The foundation for modern frameworks like React, Vue, and Angular
Master JavaScript with Cyprus's Premier Frontend Development Course
In today's digital economy, JavaScript skills are in higher demand than ever before. Our JavaScript Essentials course is designed to equip you with the programming fundamentals and practical skills needed to create dynamic, interactive websites and web applications.
The tech landscape in Cyprus continues to evolve rapidly, with more companies seeking skilled frontend developers who can build engaging user experiences. JavaScript is the cornerstone of modern web development, powering everything from simple interactive elements to complex single-page applications.
Our comprehensive 10-week program goes beyond basic syntax to teach you modern JavaScript practices, including ES6+ features, asynchronous programming, and API integration. We focus on real-world applications and problem-solving techniques that prepare you for the challenges you'll face as a professional developer.
What sets our JavaScript course apart is our hands-on, project-based approach. Instead of simply teaching theory, we guide you through building actual web applications from scratch. By the end of the course, you'll have created a portfolio-ready project that demonstrates your skills to potential employers.
Our instructors bring years of industry experience to the classroom, providing insights and best practices that go beyond what you'll find in books or online tutorials. They're committed to your success and are available for questions and guidance throughout your learning journey.
Whether you're looking to advance your career in web development, add valuable programming skills to your existing expertise, or prepare for learning advanced frameworks, our JavaScript Essentials course provides the solid foundation you need. Join us and become part of Cyprus's growing tech community!
Ready to Learn JavaScript?
Enroll in our JavaScript Essentials course today and take the next step toward becoming a professional frontend developer.